ドキュメントを参考にflutterfire_cliを使ってFlutterでFirebaseを使えるところまで進めていきます。
Firebase CLIのインストール
インストール
$ curl -sL https://firebase.tools | bash
「? Allow Firebase to collect CLI usage and error reporting information?(Firebase が CLI の使用状況やエラー報告情報を収集することを許可しますか?)」と聞かれるのでどちらかにチェック。
Firebaseのログイン確認
$ firebase login
firebaseのアカウントにログインする。
プロジェクト一覧の確認
$ firebase projects:list
firebaseのアカウントに紐づいているプロジェクト一覧を出力してくれる。
FlutterFire CLI をインストール
$ dart pub global activate flutterfire_cli
任意のディレクトリで次のコマンドを実行
Warning: Pub installs executables into $HOME/.pub-cache/bin, which is not on your path.
You can fix that by adding this to your shell's config file (.bashrc, .bash_profile, etc.):
export PATH="$PATH":"$HOME/.pub-cache/bin"
Activated flutterfire_cli 0.2.4.
警告文が表示されました。
そのままflutterfireを使うとエラーが出ます。
fish: Unknown command: flutterfire
自分の場合はfishを使っているので、PATHを通す必要があります。
$ fish_add_path $HOME/.pub-cache/bin
これでflutterfire configure
通るようになりました。
Flutterのアプリ作成
$ flutter create my_app
$ cd my_app
$ flutter run
FlutterFire CLI を使用する
$ flutterfire configure
flutterのディレクトリで実行する
$ flutterfire configure
i Found 6 Firebase projects.
✔ Select a Firebase project to configure your Flutter application with · <create a new project>
✔ Enter a project id for your new Firebase project (e.g. my-cool-project) · <project-name>
i New Firebase project <project-name> created successfully.
FirebaseCommandException: An error occured on the Firebase CLI when attempting to run a command.
COMMAND: firebase projects:create <project-name> --json
ERROR: Failed to create project because there is already a project with ID <project-name>. Please try again with a unique project ID.
create a new projectで新規に作成しようとしましたが、プロジェクト名が重複しているとエラーが出たので、プロジェクトの作成はFirebase Consoleで行いました。
Firebase Consoleの方はプロジェクト名が重複してると一意のProject IDにしてくれます。
? Which platforms should your configuration support (use arrow keys & space to select)? ›
✔ android
✔ ios
macos
web
再度$ flutterfire configure
をして、新しく作ったプロジェクトを選択すると、どのプラットフォームをサポートしますかと聞かれるので、androidとiosを選択する。
? The files android/build.gradle & android/app/build.gradle will be updated to apply Firebase configuration and gradle build plugins. Do you want to continue? (y/n) › yes
android/build.gradle & android/app/build.gradleのファイルは、Firebase の設定と gradle build プラグインを適用するために更新します。yes or noと聞かれるので、yesを選択する。
❯ flutterfire configure
i Found 7 Firebase projects.
✔ Select a Firebase project to configure your Flutter application with · xxx (xxx)
✔ Which platforms should your configuration support (use arrow keys & space to select)? · android, ios
i Firebase android app com.example.xxx is not registered on Firebase project xxx.
i Registered a new Firebase android app on Firebase project xxx.
i Firebase ios app com.example.xxx is not registered on Firebase project xxx.
i Registered a new Firebase ios app on Firebase project xxx.
✔ The files android/build.gradle & android/app/build.gradle will be updated to apply Firebase configuration and gradle build plugins. Do you want to continue? · yes
Firebase configuration file lib/firebase_options.dart generated successfully with the following Firebase apps:
Platform Firebase App Id
android xxx
ios xxx
Learn more about using this file and next steps from the documentation:
> https://firebase.google.com/docs/flutter/setup
無事に完了しました。
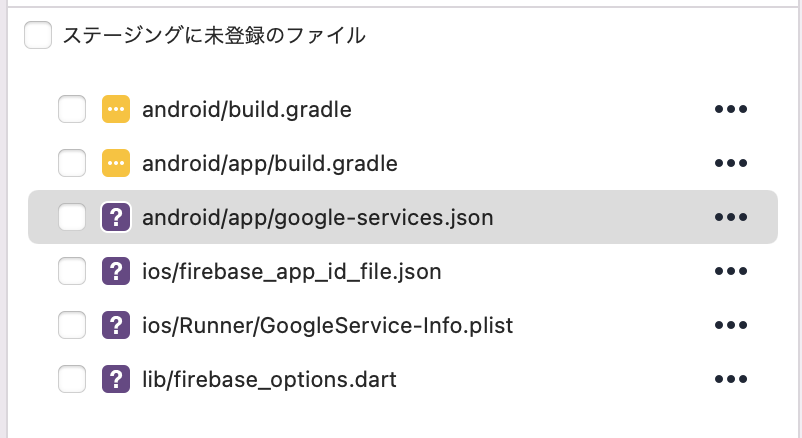
Gitの差分。
Firebase上のアプリの追加もコンソール上でできるのが楽ですね。
今まではコンソール上で色々設定した後にgoogle-services.jsonをダウンロードしてたのが、かなり楽になりました。
注意事項
flutterfire configure
の初回実行後、以下の場合には必ず、コマンドを再実行する必要があります。– Flutter アプリで新しいプラットフォームをサポートする場合。
– Flutter アプリで新しい Firebase サービスまたはプロダクトの使用を開始する場合。特に Google、Crashlytics、Performance Monitoring、Realtime Database でログインの使用を開始する場合。コマンドを再実行すると、Flutter アプリの Firebase 構成が最新の状態になり、(Android の場合)必要な Gradle プラグインがアプリに自動的に追加されます。
https://firebase.google.com/docs/flutter/setup?platform=ios
注意事項で上記のように記載がありました。
新しくプラットフォームを追加する場合や、Firebaseのサービスの使用開始する場合はflutterfire configure
を再度実行する必要がありそうです。
アプリでFirebaseを初期化する
$ flutter pub add firebase_core
パッケージfirebase_coreをインストールするlib/firebase_options.dart
で表示されていたWarningも無くなりました。
$ flutterfire configure
Firebaseの構成を最新にします。
// lib/main.dart
import 'package:firebase_core/firebase_core.dart';
import 'firebase_options.dart';
main.dartに上記をインポートする。
await Firebase.initializeApp(
options: DefaultFirebaseOptions.currentPlatform,
);
Firebaseを初期化します。
// コード全体
void main() async {
// Firebase.initializeAppの前に必要。
WidgetsFlutterBinding.ensureInitialized();
await Firebase.initializeApp(
options: DefaultFirebaseOptions.currentPlatform,
);
runApp(const MyApp());
}
コード全体です。
上記の書き方はflutterfireのドキュメントを参考にしました。
他にも初期化の方法はいくつかあるようです。
$ flutter run
再度ビルドします。
これでFlutterでFirebaseを使う準備はできました。
Firebase プラグインを追加する
Firebaseの各種プラグインを使う場合は以下のように行います。
$ flutter pub add PLUGIN_NAME
Firebaseのプラグインを入れる。
使用できるプラグイン一覧。
$ flutterfire configure
Firebaseの構成を最新の状態にする。
$ flutter run
再度ビルドする。
これで完了です。